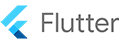
Flutter Track Training
`Flutter in one month training plan
Week 1: Flutter Fundamentals
Day 1-2: Setup and Introduction
- Installing Flutter SDK, IDE setup (VSCode/Android Studio).
- Creating your first Flutter project.
- Overview of Dart language basics (variables, functions, loops, classes).
- Hands-on: Simple "Hello World" app.
Day 3-4: Basic Widgets
- Core Flutter widgets (Text, Image, Button, Row, Column, Stack).
- Layout basics and the use of Container, Padding, SizedBox, and Align.
- Hands-on: Build a static UI screen using core widgets (like a profile page or product display).
Day 5: Input and Gesture Handling
- TextInput (TextField), Buttons (RaisedButton, FlatButton), handling taps and gestures.
- Hands-on: Build a basic form with input fields and buttons (e.g., login form or survey form).
Day 6-7: Introduction to Stateful Widgets
- Difference between stateless and stateful widgets.
- Managing state using setState.
- Hands-on: Build a counter app (with buttons to increment/decrement values).
Week 2: Layouts, Navigation, and Lists
Day 1-2: Flutter Layouts Deep Dive
- Advanced layouts using Flex, Expanded, and ListView.
- Hands-on: Build a simple app with multiple screens using Column, Row, Expanded, and ListView.
Day 3-4: Navigation and Routing
- Navigating between screens using Navigator.push and Navigator.pop.
- Named routes and passing data between screens.
- Hands-on: Build a simple navigation app (e.g., Home > Details screen).
Day 5: Lists and Grids
- Displaying lists using ListView.builder and GridView.
- Scrollable widgets, using the ListTile widget.
- Hands-on: Build a product list page with a grid or list view.
Day 6-7: Simple State Management
- Introduction to basic state management using InheritedWidget and Provider (without Bloc or complex solutions).
- Managing global state across multiple widgets.
- Hands-on: Build a to-do list app where tasks can be added and marked as done using Provider.
Week 3: Forms, Validation, and Animation
Day 1-2: Working with Forms
- Handling forms in Flutter (Form, TextFormField).
- Input validation and form submission.
- Hands-on: Build a registration or login form with validation (email, password fields).
Day 3-4: Introduction to Animations
- Implicit animations (AnimatedContainer, AnimatedOpacity).
- Explicit animations using AnimationController and Tween.
- Hands-on: Create simple animations (e.g., fade in/out, size transitions).
Day 5: Dialogs, Snackbars, and Bottom Sheets
- Showing dialogs, bottom sheets, and snackbars.
- Handling user interactions with these components.
- Hands-on: Build a settings screen where a user can trigger dialogs and snackbars.
Day 6-7: Media and Assets
- Working with images, icons, and other assets.
- Adding and using local assets (images, fonts).
- Hands-on: Build a gallery or photo viewer app.
Week 4: Project and Final Concepts
Day 1-2: Theme and Customization
- Introduction to themes, color schemes, and global styles.
- Applying light and dark themes.
- Hands-on: Theme a basic app (e.g., changing color schemes and text styles).
Day 3-4: Introduction to Networking (Optional)
- (Basic API calls only for practice, optional)
- Fetching data from an API using http package.
- Displaying fetched data in a list.
- Hands-on: Build a news or weather app (if networking is needed).
Day 5-7: Final Project
- Capstone project: Build a simple, functional app that combines everything learned (e.g., a basic shopping list, to-do app, or movie catalog).
- Focus on UI, navigation, state management, and simple animations.