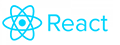
React One Month Training Plan
Week 1: React Fundamentals
Day 1-2: Setup and Introduction.
- Installing Node.js, npm (Node Package Manager), and Create React App.
- Creating your first React project and running it locally.
- Introduction to React components, JSX syntax, and basic structure.
Hands-on:
Create a simple "Hello World" React app that displays a greeting.
Day 3-4: Basic React Components and JSX
- Understanding React components (functional and class components).
- Introduction to JSX (embedding JavaScript within HTML-like syntax).
- How to render content dynamically using props.
Hands-on:
Build a component that displays user information (name, age, etc.) passed as props.
Day 5: State and Event Handling
- Introduction to useState for managing component state.
- Handling user events like button clicks and form submissions.
- Using input in simple programs.
Hands-on:
Create a counter app with increment and decrement buttons using useState.
Day 6-7: Introduction to React's Functional Components.
- Introduction to functional components and hooks.
- Understanding the lifecycle of functional components with useEffect.
Hands-on:
Build a simple todo list app where tasks can be added and deleted using functional components.
Week 2: Layouts, Routing, and Lists
Day 1-2: React Layouts and Styling
- Understanding how to use div, section, header, and other layout elements in React.
- Using inline styles, CSS modules, and styled-components for styling.
Hands-on:
Create a simple homepage layout with a navigation bar and content area.
Day 3-4: React Router
- Introduction to React Router for client-side navigation.
- Setting up routes,
and components for navigation.
Hands-on:
Build a multi-page app with a homepage, about page, and contact page using React Router.
Day 5: Lists and Dynamic Rendering
- Rendering lists using map() and dynamic content with React.
- Using keys in lists for performance optimization.
Hands-on:
Create a product catalog page where products are displayed in a list or grid layout.
Day 6-7: State Management (useContext & useReducer)
- Introduction to React's Context API and useContext for managing global state.
- Using useReducer as an alternative for more complex state management.
Hands-on:
Build a simple shopping cart app using useContext to manage the cart state across components.
Week 3: Forms, Validation, and Animations
Day 1-2: Working with Forms
- Handling user input with input, textarea, select, and form components in React.
- Managing form data with useState and controlled components.
- Form validation and submission handling.
Hands-on:
Create a login or registration form with field validation (e.g., email, password).
Day 3-4: Animations and Transitions
- Introduction to animations in React with libraries like react-spring or framer-motion.
- Animating components on mount, unmount, and interactions.
Hands-on:
Create a fade-in animation for a component when it’s first rendered or clicked.
Day 5: Modals, Alerts, and Notifications
- Building custom modal components in React.
- Showing alert messages and notifications (using libraries like react-toastify).
Hands-on:
Build a settings page with a modal dialog for user preferences.
Day 6-7: Working with Media and Assets
- Handling images, fonts, and other assets in React.
- Importing and using static assets in your React app (e.g., local images or icons).
Hands-on:
Build a gallery or image viewer app with images loaded from static assets.
Week 4: Project and Final Concepts
Day 1-2: Customizing Themes and Global Styles
- Introduction to theming in React (light and dark themes).
- Managing global styles and themes using styled-components or CSS variables.
Hands-on:
Apply custom themes to an existing app (e.g., toggle between light and dark mode).
Day 3-4: Fetching Data (Optional)
- Making API requests with fetch or axios to get data from external APIs.
- Handling API responses and displaying data in a list.
Hands-on:
Build a weather app that fetches data from a public API (optional if networking is required).
Day 5-7: Final Project
- Capstone project:
Create a fully functional React app that combines everything you've learned. - Example apps:
a movie catalog, task manager, or shopping list. - Focus on components, routing, state management, and basic animations.
- Implement features like forms, API calls (if applicable), and dynamic content.
Learning Outcomes By the end of this month, you will be able to:
- Build responsive, dynamic web apps using React.
- Manage application state with hooks like use State and use Context.
- Handle routing and navigation in React apps with React Router.
- Build interactive forms, animations, and manage media assets.
- Deploy a React app to production and create a fully functional app from scratch.